In the previous lesson, you saw some example code for creating a new driver instance.
Let’s take a closer look at the driver()
method and how it is used to create a driver instance.
const driver = neo4j.driver(
connectionString, // (1)
authenticationToken, // (2)
configuration // (3)
)
The neo4j.driver()
function accepts the following arguments:
-
A connection string
-
An authentication token
-
Optionally, an object containing additional driver configuration
Let’s take a look at these arguments in more detail.
1
The Connection String
A connection string typically consists of four elements:
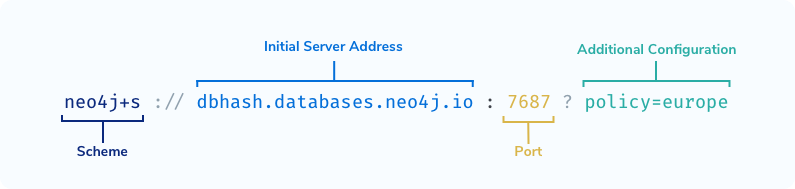
-
The scheme used to connect to the Neo4j instance - for example
neo4j
orneo4j+s
(required) -
The initial server address for the Neo4j DBMS - for example
localhost
ordbhash.databases.neo4j.io
(required) -
The port number that the DBMS is running on (required if the instance is not running on the default port of
7687
) -
Additional connection configuration (for example the routing context)
Choosing your Scheme
Most likely, you will use a variation of the neo4j
scheme within your connection string.
-
neo4j
- Creates an unencrypted connection to the DBMS. If you are connecting to a local DBMS or have not explicitly turned on encryption then this is most likely the option you are looking for. -
neo4j+s
- Creates an encrypted connection to the DBMS. The driver will verify the authenticity of the certificate and fail to verify connectivity if there is a problem with the certificate. -
neo4j+ssc
- Creates an encrypted connection to the DBMS, but will not attempt to verify the authenticity of the certificate.
Variations of the bolt
scheme can be used to connect directly to a single DBMS (within a clustered environment or standalone). This can be useful if you have a single server configured for data science or analytics.
-
bolt
- Creates an unencrypted connection directly to a single DBMS. -
bolt+s
- Creates an encrypted connection directly to a single DBMS and verify the certificate. -
bolt+ssc
- Creates an encrypted connection to directly to a single DBMS but will not attempt to verify the authenticity of the certificate.
Which scheme string is right for you?
You can verify the encryption level of your DBMS by checking the dbms.connector.bolt.enabled
key in neo4j.conf
.
If you are connecting to a DBMS hosted on Neo4j Aura, you will always use the neo4j+s
scheme.
Additional Connection Information
Additional connection information can be appended to the connection string after a ?
.
For example, in a multi-data centre cluster, you may wish to take advantage of locality to reduce latency and improve performance.
In this case, you could configure a routing policy for a set of application servers to connect to Neo4j instances that are within the same data centre.
For more information, see this Knowledge Base Article: Consideration about routing tables on multi-data center deployments.
2
An Authentication Token
In most cases, you will connect to the DBMS using basic authentication consisting of a username and password.
You can create a basic authentication token by calling the basic()
method on the neo4j.auth
object.
neo4j.auth.basic(username, password)
For more information on additional authentication methods, see the Authentication and authorization section of the Neo4j Operations Manual.
3
Additional Driver Configuration (Optional)
The driver()
method also accepts a third additional configuration object.
This object allows you to provide advanced configuration options, for example setting the connection pool size or changing timeout limits.
{
maxConnectionPoolSize: 100,
connectionTimeout: 30000, // 30 seconds
logging: {
level: 'info',
logger: (level, message) => console.log(level + ' ' + message)
},
}
For more information or a full list of configuration options, please visit the Neo4j JavaScript Driver manual.
What happens next?
The driver will attempt to connect to the DBMS using the supplied credentials. If everything is successful, the driver will then communicate with the DBMS and figure out the best way to execute each query.
You do not need to do any additional configuration when connecting to a single DBMS or a Neo4j cluster. This means you do not have to adapt your application code, regardless of which environment you connect to.
Once the connection has been successfully made, your application can start to interact with the data in the graph.
Check Your Understanding
1. Which of the following options are valid schemes to use in the connection string?
-
❏
aura://
-
✓
bolt://
-
❏
graphdb://
-
✓
neo4j://
-
✓
neo4j+s://
Hint
There are three valid options above.
Solution
From the options above, both neo4j://
and neo4j+s://
are schemes used for either connecting to a single instance or cluster without needing to change configuration, while bolt://
for creating a direct connection to a specific Neo4j server=.
2. Which scheme should you use when connecting to a Neo4j Aura Database?
-
❏
aura://
-
❏
bolt://
-
❏
neo4j://
-
✓
neo4j+s://
Hint
A secure connection is required to a Neo4j Aura database.
Solution
The correct answer is neo4j+s://
.
Lesson Summary
In this lesson, you learned how to configure the driver.
In the next Challenge, you will add a Driver instance to the project.