This challenge has three parts:
The My Favorites Feature
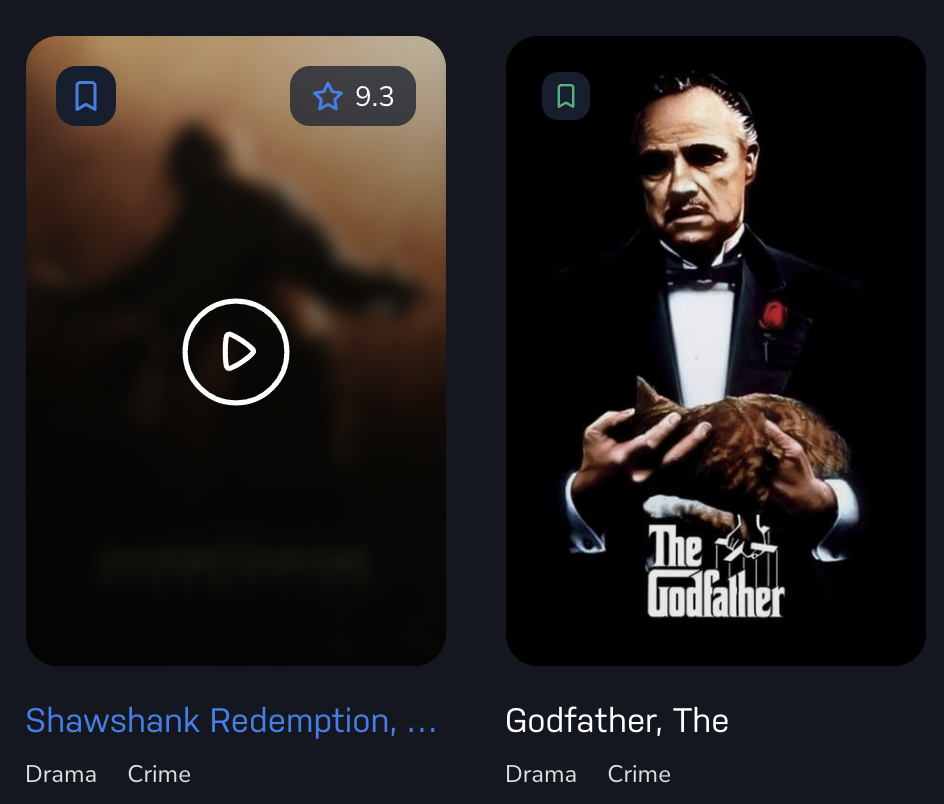
Clicking the My Favorites link in the main navigation will take you to page which contains a list of Movies that you can revisit later.
When a logged in user hovers their mouse cursor over a Movie on the website, a bookmark icon appears in the top right hand corner. Clicking this icon will either add or remove the Movie from the user’s Favorites list.
When adding a Movie to the list, a POST
request it sent to the /api/favorites/{id}
endpoint. When this happens, the following chain of events will occur:
-
The server directs the request to the route handler in
src/routes/account.routes.js
, which verifies the user’s JWT token before handling the request -
The route handler creates an instance of the
FavoriteService
. -
The
add()
method is then called on theFavoriteService
instance with the user’s ID taken from the JWT token, and the ID of the movie that has been extracted from the request URL. -
It is then the responsibility of the
FavoriteService
to find the:User
and:Movie
nodes and create the:HAS_FAVORITE
relationship between them.
Likewise, when it is clicked for a Movie that has already been favorited, a DELETE
request is sent to the same URL, and the Movie is removed from the list.
Adding a Movie to My Favorites
For the first part of this challenge, modify the add()
method to open a new database session, run the Cypher statement to create the :HAS_FAVORITE
relationship, close the session and return the movie details along with an additional favorite
property.
src/services/favorites.service.js
→
1. Open the Session
Call the session()
method on the Driver instance to open a new session:
Unresolved directive in lesson.adoc - include::https://raw.githubusercontent.com/neo4j-graphacademy/llm-vectors-unstructured/main/example/index.js[tag=session]
2. Create the Relationship
In a write transaction, run a Cypher statement to create the :HAS_RELATIONSHIP
relationship between the User
and Movie
nodes, with a createdAt
property to represent when the relationship was created.
Click here to reveal the Cypher statement
MATCH (u:User {userId: $userId})
MATCH (m:Movie {tmdbId: $movieId})
MERGE (u)-[r:HAS_FAVORITE]->(m)
ON CREATE SET r.createdAt = datetime()
RETURN m {
.*,
favorite: true
} AS movie
Unresolved directive in lesson.adoc - include::https://raw.githubusercontent.com/neo4j-graphacademy/llm-vectors-unstructured/07-favorites-list/src/services/favorite.service.js[tag="create"]
3. Does the Movie exist?
If no records are returned, you can safely assume that the either the User or Movie do not exist.
In this case, throw a NotFoundError
with an appropriate error message.
Unresolved directive in lesson.adoc - include::https://raw.githubusercontent.com/neo4j-graphacademy/llm-vectors-unstructured/07-favorites-list/src/services/favorite.service.js[tag="throw"]
4. Close the Session
Before the movie information information is returned, ensure that the session is closed.
Unresolved directive in lesson.adoc - include::https://raw.githubusercontent.com/neo4j-graphacademy/llm-vectors-unstructured/main/example/index.js[tag="session.close"]
5. Return Results
Then, finally, use the toNativeTypes()
function to return the movie
value from the first record returned from the database.
Unresolved directive in lesson.adoc - include::https://raw.githubusercontent.com/neo4j-graphacademy/llm-vectors-unstructured/07-favorites-list/src/services/favorite.service.js[tag="return",indent=0]
Working Solution
Click here to reveal the completed add()
method
Unresolved directive in lesson.adoc - include::https://raw.githubusercontent.com/neo4j-graphacademy/llm-vectors-unstructured/07-favorites-list/src/services/favorite.service.js[tag=add]
Removing a Movie from My Favorites
The second part of this challenge is to write the code to remove a movie from the My Favorites list.
The code for deleting the :HAS_FAVORITE
relationship will be similar, only the Cypher statement will change.
Instead of two separate MATCH
clauses, we can instead attempt to find the pattern within a single clause.
If the relationship (with an alias of r
) exists, we will delete it and then return the movie information with favorite
set to false
.
MATCH (u:User {userId: $userId})-[r:HAS_FAVORITE]->(m:Movie {tmdbId: $movieId})
DELETE r
RETURN m {
.*,
favorite: false
} AS movie
Use the code from the add()
method above to implement the remove()
function.
If you get stuck, you can reveal the completed method below.
Working Solution
Click here to reveal the completed remove()
method
Unresolved directive in lesson.adoc - include::https://raw.githubusercontent.com/neo4j-graphacademy/llm-vectors-unstructured/07-favorites-list/src/services/favorite.service.js[tag=remove]
Listing My Favorites
Finally, the all()
method in the FavoriteService
currently returns a hardcoded list of Movies.
Unresolved directive in lesson.adoc - include::https://raw.githubusercontent.com/neo4j-graphacademy/llm-vectors-unstructured/main/src/services/favorite.service.js[tag=all]
Update this method to return a paginated list of movies that the user has added to their My Favorites list.
Click here to reveal the Cypher statement
MATCH (:User {userId: $userId})-[:HAS_FAVORITE]->(m)
RETURN m {
.*,
favorite: true
} AS movie
You have already written similar code a few times, so try to implement this one on your own. If you get stuck, you can view the full solution below.
Remember to also import the int()
function from neo4j-driver
.
This is required to convert the skip
and limit
values to Neo4j integers.
Click here to reveal the completed all()
method
Unresolved directive in lesson.adoc - include::https://raw.githubusercontent.com/neo4j-graphacademy/llm-vectors-unstructured/07-favorites-list/src/services/favorite.service.js[tag=all]
Testing
To test that this functionality has been correctly implemented, run the following code in a new terminal session:
npm run test 07
The test file is located at test/challenges/07-favorites-list.spec.js
.
Are you stuck? Click here for help
If you get stuck, you can see a working solution by checking out the 07-favorites-list
branch by running:
git checkout 07-favorites-list
You may have to commit or stash your changes before checking out this branch. You can also click here to expand the Support pane.
Verifying the Test
If the test has run successfully, a user with the email address graphacademy.favorite@neo4j.com
will have added the movie Toy Story to their list of favorites.
Hint
You can run the following query to check for the user within the database.
If the shouldVerify
value returns true, the verification should be successful.
MATCH (u:User {email: "graphacademy.favorite@neo4j.com"})-[:HAS_FAVORITE]->(:Movie {title: 'Toy Story'})
RETURN true AS shouldVerify
Solution
The following statement will mimic the behaviour of the test, merging a new :User
node with the email address graphacademy.favorite@neo4j.com
and ensuring that a node exists for the movie Toy Story.
The test then merges a :HAS_FAVORITE
relationship between the user and movie nodes.
MERGE (u:User {userId: '9f965bf6-7e32-4afb-893f-756f502b2c2a'})
SET u.email = 'graphacademy.favorite@neo4j.com'
MERGE (m:Movie {tmdbId: '862'})
SET m.title = 'Toy Story'
MERGE (u)-[r:HAS_FAVORITE]->(m)
RETURN *
Once you have run this statement, click Try again…* to complete the challenge.
Module Summary
In this Challenge, you have written the code to find or create a :HAS_FAVORITE
relationship between a User
and a Movie
within a write transaction.
In the next Challenge, you will write code to execute multiple queries in the same transaction.