Property graph
In the previous module we referred to nodes and relationships as the fundamental building blocks for a graph. In this lesson you will learn about the additional elements that Neo4j supports to make a property graph.
Nodes, Labels and Properties
Recall that nodes are the graph elements that represent the things in our data. We can use two additional elements to provide some extra context to the data.
Let’s take a look at how we can use these additional elements to improve our social graph.
Labels
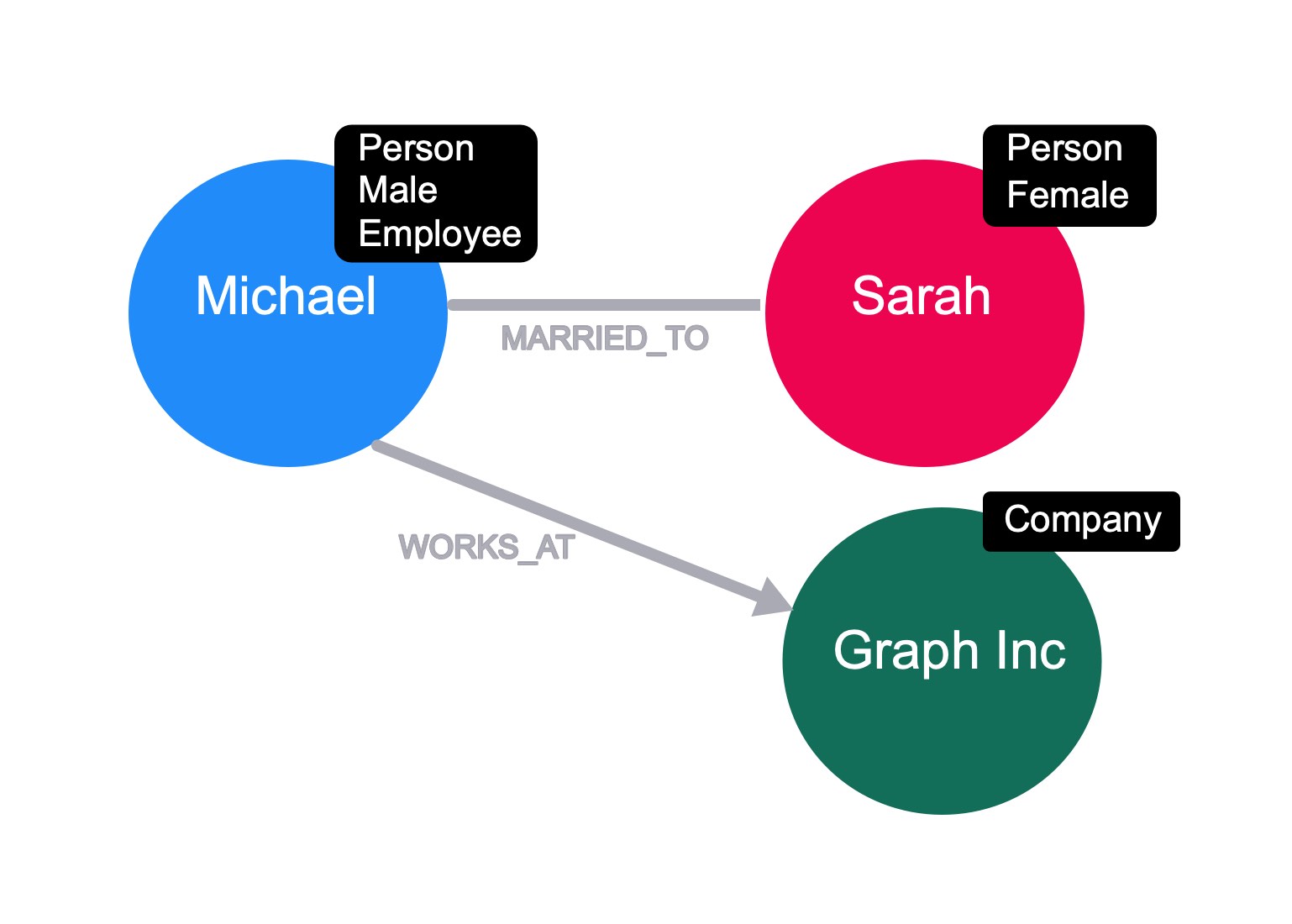
By adding a label to a node, we are signifying that the node belongs to a subset of nodes within the graph. Labels are important in Neo4j because they provide a starting point for a Cypher statement.
Let’s take Michael and Sarah - in this context both of these nodes are persons.
We can embellish the graph by adding more labels to these nodes; Michael identifies as male and Sarah is female. In this context, Michael is an employee of a company, but we don’t have any information about Sarah’s employment status.
Michael works for a company called Graph Inc, so we can add that label to the node that represents a company.
Node properties
So far we’re assuming that the nodes represent Michael, Sarah, and Graph Inc. We can make this concrete by adding properties to the node.
Properties are key, value pairs and can be added or removed from a node as necessary. Property values can be a single value or list of values that conform to the Cypher type system.
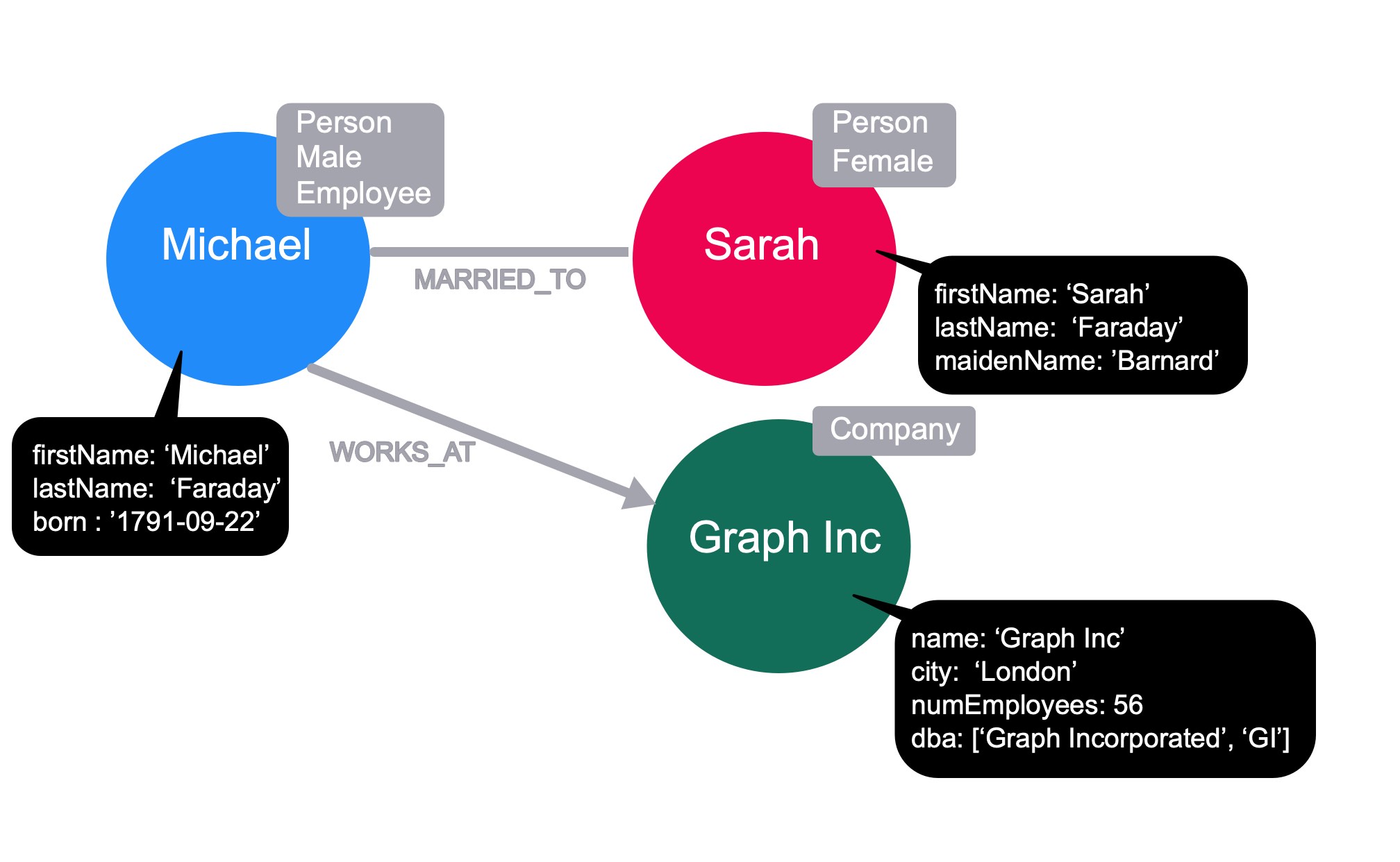
By adding firstName and lastName properties, we can see that the Michael node refers to Michael Faraday, known for Faraday’s law of induction, the Faraday cage and lesser known as the inventor of the Party Balloon. Michael was born on 22 September 1791.
Sarah’s full name is Sarah Faraday, and her maidenName is Barnard.
By looking at the name property on the Graph Inc node, we can see that it refers to the company Graph Inc, with a city of London, has 56 employees (numEmployees), and does business as Graph Incorporated and GI (dba).
null
value.Relationships
A relationship in Neo4j is a connection between two nodes.
Relationship direction
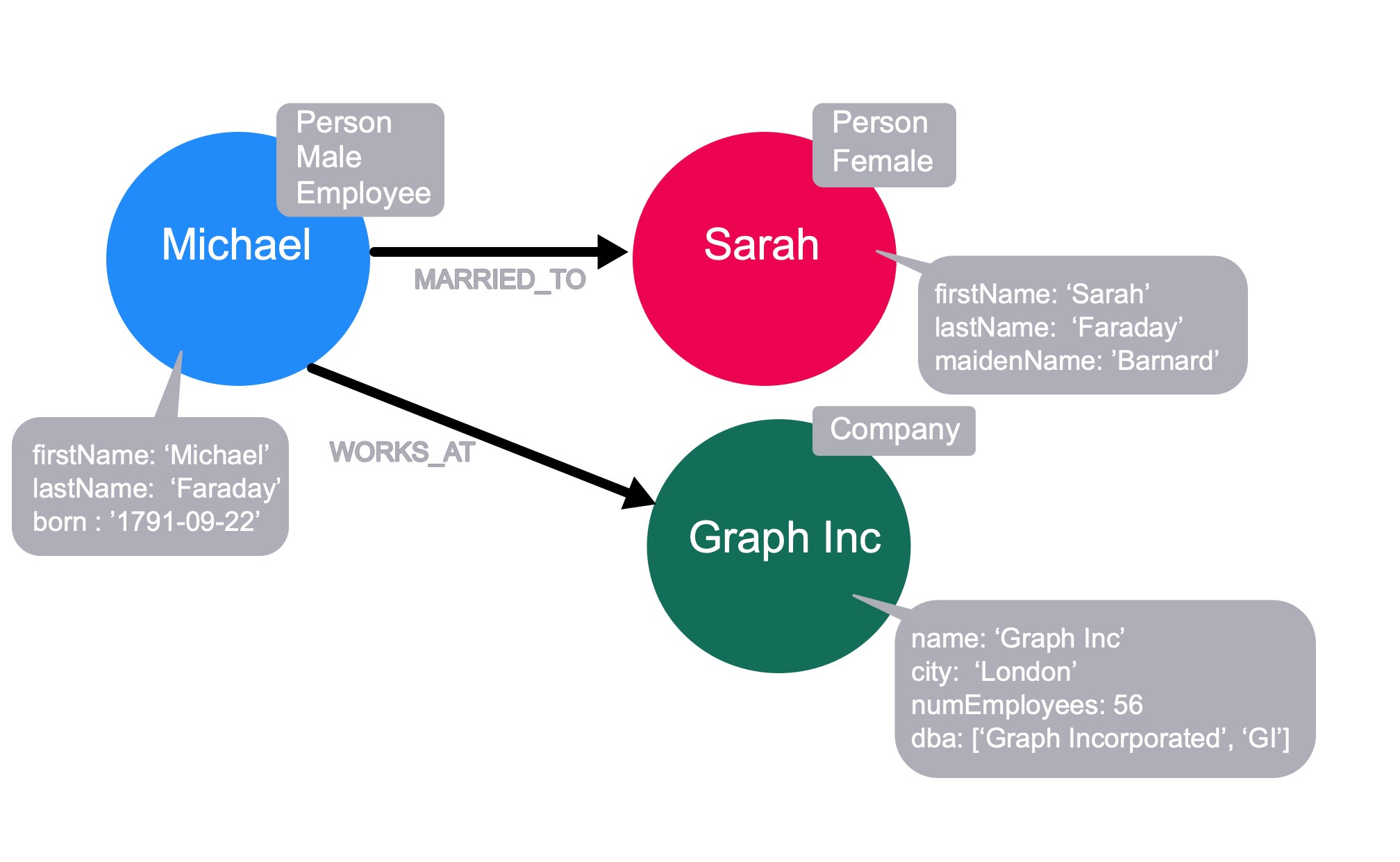
In Neo4j, each relationship must have a direction in the graph. Although this direction is required, the relationship can be queried in either direction, or ignored completely at query time.
A relationship is created between a source node and a destination node, so these nodes must exist before you create the relationship.
If we consider the concept of directed & undirected graphs that we discussed in the previous module, the direction of the MARRIED_TO relationship must exist and may provide some additional context but can be ignored for the purpose of the query. In Neo4j, the MARRIED_TO relationship must have a direction.
The direction of a relationship can be important when it comes to hierarchy, although whether the relationships point up or down towards the tree is an arbitrary decision.
Relationship type
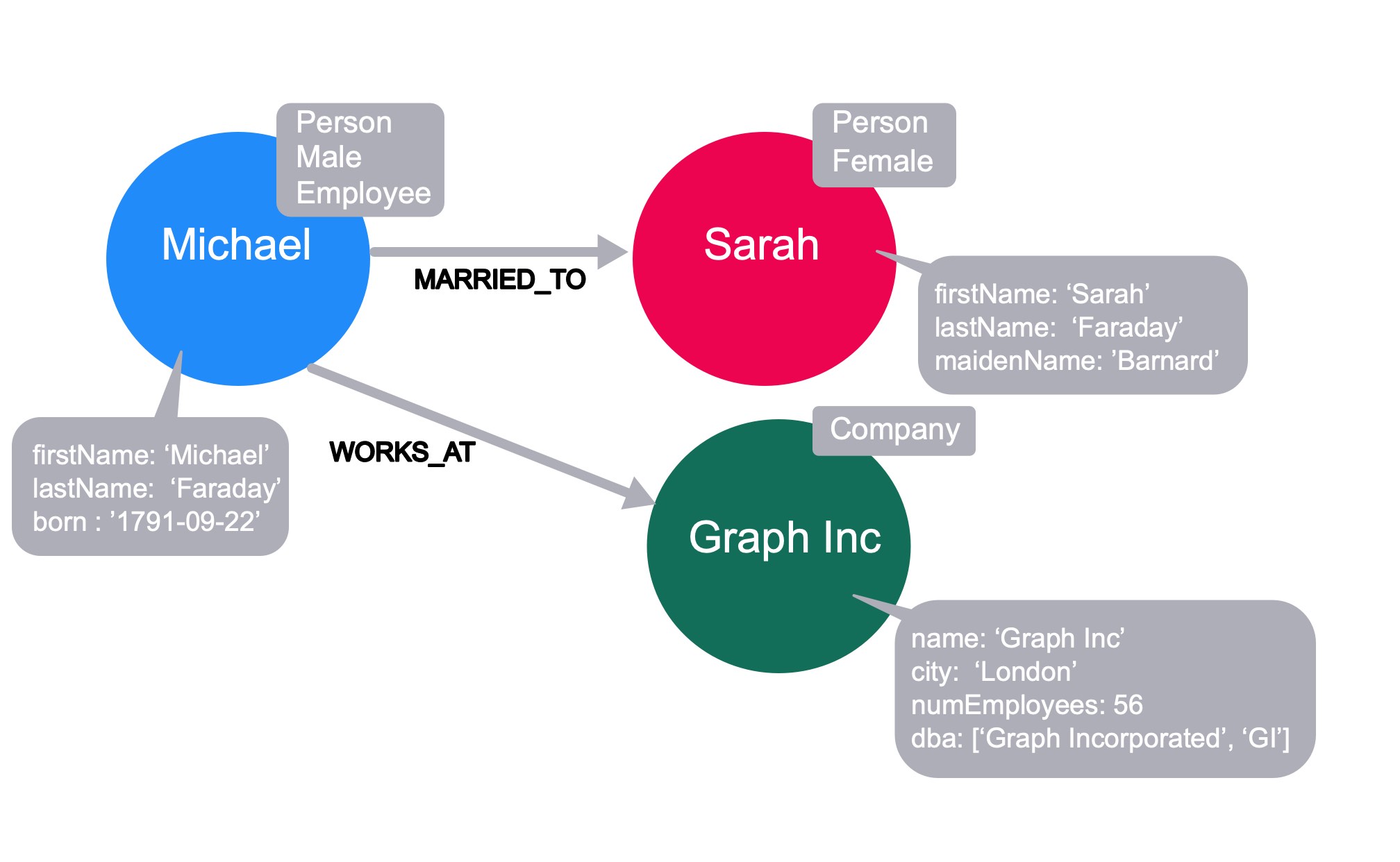
Each relationship in a neo4j graph must have a type. This allows us to choose at query time which part of the graph we will traverse.
For example, we can traverse through every relationship from Michael, or we can specify the MARRIED_TO relationship to end up only at Sarah’s node.
Here are sample Cypher statement statements to support this:
// traverse the Michael node to return the Sarah node
MATCH (p:Person {firstName: 'Michael'})-[:MARRIED_TO]-(n) RETURN n;
// traverse the Michael node to return the Graph Inc node
MATCH (p:Person {firstName: 'Michael'})-[:WORKS_AT]-(n) RETURN n;
// traverse all relationships from the Michael node
// to return the Sarah node and the Graph Inc node
MATCH (p:Person {firstName: 'Michael'})--(n) RETURN n
Relationship properties
As with nodes, relationships can also have properties. These can refer to a cost or distance in a weighted graph or just provide additional context to a relationship.
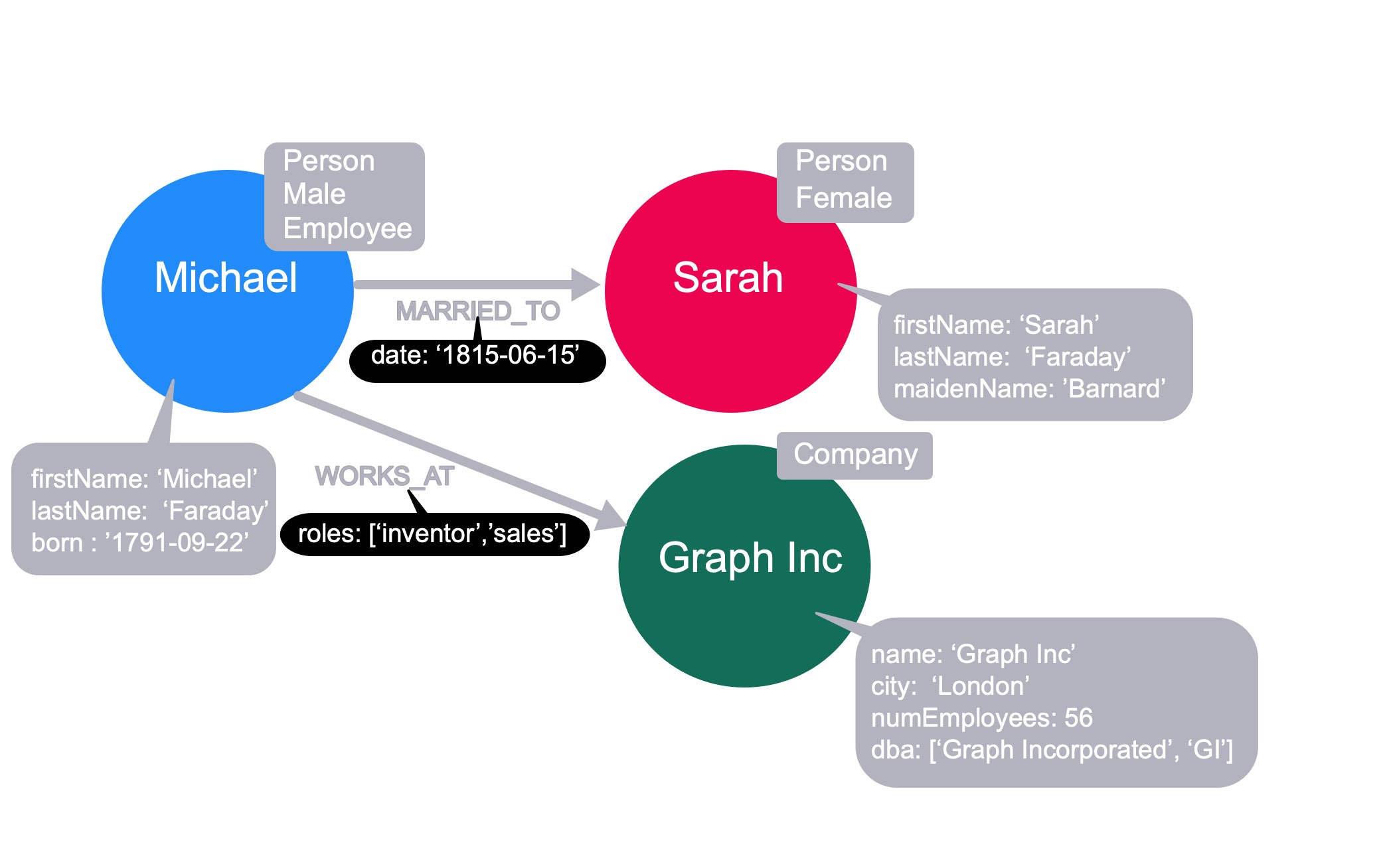
In our graph, we can place a property on the MARRIED_TO relationship to hold the date in which Michael and Sarah were married. This WORKS_AT relationship has a roles property to signify any roles that the employee has filled at the company. If Michael also worked at another company, his WORKS_AT relationship to the other company would have a different value for the roles property.
Check your understanding
1. Nodes in Neo4j
What two elements do you add to a node to make it represent the entities of your domain?
-
❏ direction
-
✓ label
-
❏ type
-
✓ properties
Hint
You use one of these elements to categorize the nodes in the graph and you use one of these elements to describe the data for a node.
Solution
You add a label and properties to represent the entities of your domain.
2. Relationships in Neo4j
What must relationships in Neo4j have?
-
❏ a property
-
❏ a weight
-
✓ a type
-
✓ a direction
Hint
All relationships in Neo4j must have these two things.
Solution
A relationship must have a from and to node, direction and a type.
Summary
In this lesson you learned that Neo4j’s implementation of property graphs include labels for nodes and properties for both nodes and relationships. Next, you will learn why Neo4j’s implementation of a native graph provides superior performance.