So far in the course, you have explored the Query API of the Neo4j GraphQL Library. In this section, you will learn about GraphQL mutation operations that create, update, or delete nodes and their properties in the database.
In the Neo4j GraphQL Toolbox, at the bottom of the Explorer pane, select Mutation from the type dropdown and click the "+" button to add the Mutation fields to the Explorer pane.
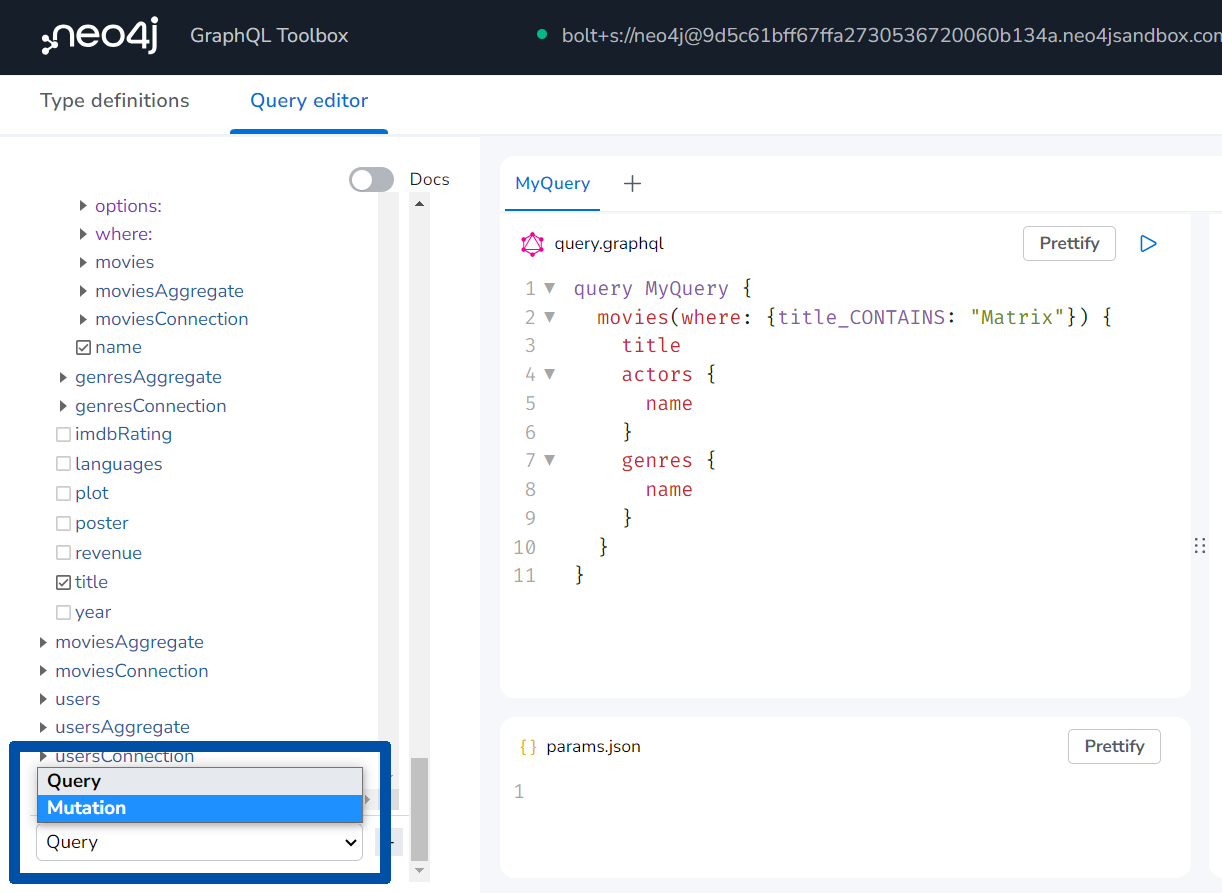
The default create, update, and delete mutation operations are generated for each type in the GraphQL schema and displayed in the query editor. Let’s see how to use these mutations!
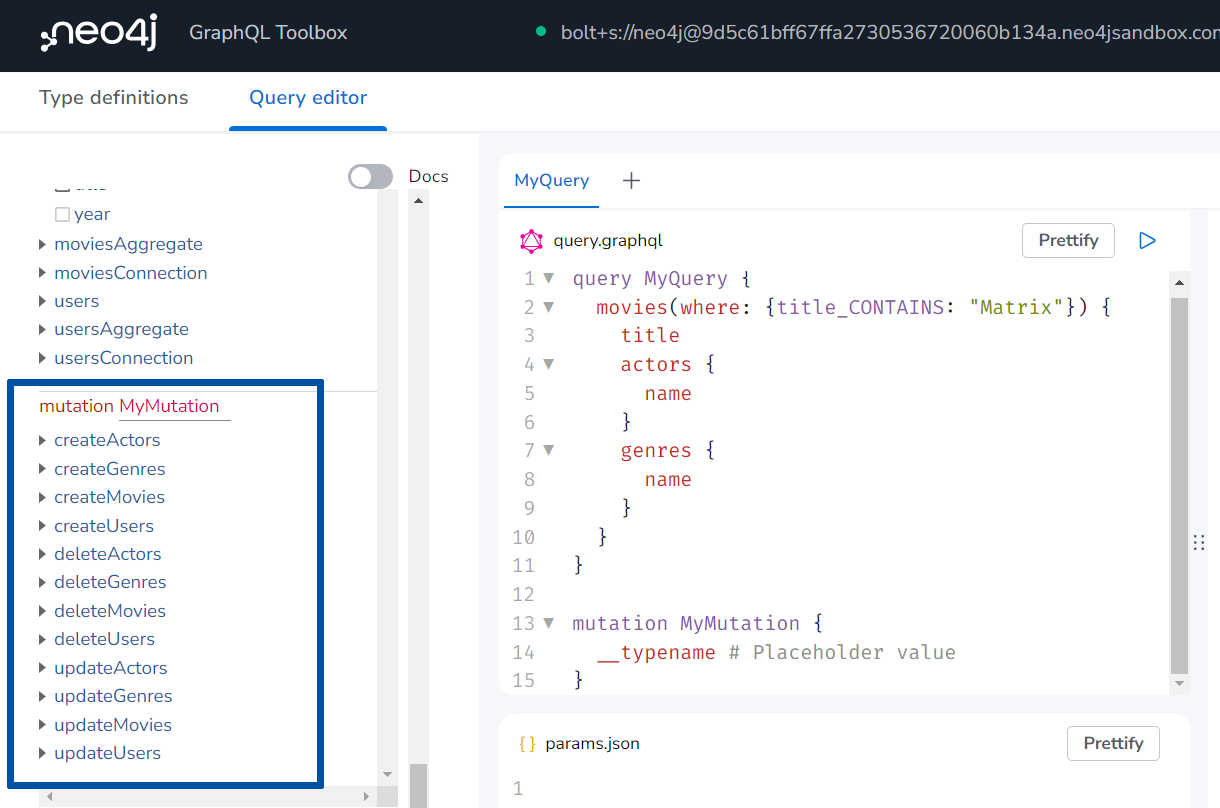
Create
First, let’s create a new Genre node to represent my favorite genre Cli-Fi or, Science Fiction focused around Climate Change.
Run the following mutation query to create a new genre.
mutation MyMutation {
createGenres(input: {name: "CliFi"}) {
genres {
name
}
}
}
The response includes the details of the new "CliFi" node which has been created in the database:
{
"data": {
"createGenres": { "genres": [{ "name": "CliFi" }] }
}
}
Oops - I misspelled "Cli-Fi"! You can use the update mutation to change the name property of this node.
Update
To filter for the node(s) you want to update - you would use a where
argument with an update mutation operation.
This query filters for the misspelled "CliFi" node, then specifies the field values to be updated.
mutation MyMutation {
updateGenres(where: { name: "CliFi" }, update: { name: "Cli-Fi" }) {
genres {
name
}
}
}
The response confirms that the name
property has been updated:
{
"data": {
"updateGenres": { "genres": [{ "name": "Cli-Fi" }] }
}
}
Delete
Similarly to the update mutation, you can use a where
argument to filter for the node(s) you want to delete with a delete mutation.
This query will delete the "Cli-Fi" node:
mutation MyMutation {
deleteGenres(where: { name: "Cli-Fi" }) {
nodesDeleted
relationshipsDeleted
}
}
The number of deleted nodes and relationships are returned in the response as nodesDeleted
and relationshipsDeleted
. You can see that one node was deleted (the Cli-Fi genre node).
{
"data": {
"deleteGenres": {
"nodesDeleted": 1,
"relationshipsDeleted": 0
}
}
}
Check Your Understanding
1. GraphQL Operations
Fill in the blank:
GraphQL ____ operations are used to create, update, or delete nodes and their properties.
-
❏ upsert
-
❏ subscription
-
✓ mutation
-
❏ query
Hint
There are three types of GraphQL operations: query, mutation, and subscription.
Solution
GraphQL mutation operations are used to create, update, or delete nodes and their properties.
2. Update and Delete Mutations
Fill in the blank:
Typically update and delete mutation operations are used with a ____ argument to filter for the nodes to be deleted or updated.
Select the correct answer.
-
✓
where
-
❏
options
-
❏
filter
-
❏
protected
Hint
You used this optional argument in the previous lesson to filter results.
Solution
You would typically use update and delete mutation operations with a where
argument to filter for the nodes to be deleted or updated.
Summary
In this lesson, you learned about some GraphQL mutation operations available with the Neo4j GraphQL Library for working with nodes.
In the next lesson, you will see how to perform mutations with relationships and more complex mutation operations with the nested mutation feature of the Neo4j GraphQL Library.