You will update a starter project to create your chatbot.
As you progress through the course, you will build the functionality to turn the Chatbot into an intelligent movie recommendation assistant. It will use the data in a Neo4j database to improve the responses generated by an LLM.
In this lesson, you will learn about the components you will work with.
Streamlit
Streamlit is an open-source Python library that allows developers to create web applications for data-centric projects with minimal effort.
With minimal code, developers and data scientists can convert data scripts into functional web applications without writing front-end-specific code. Its simple API and compatibility with existing Python-based projects and tools means seamless integration, reducing the overhead typically associated with web application development.
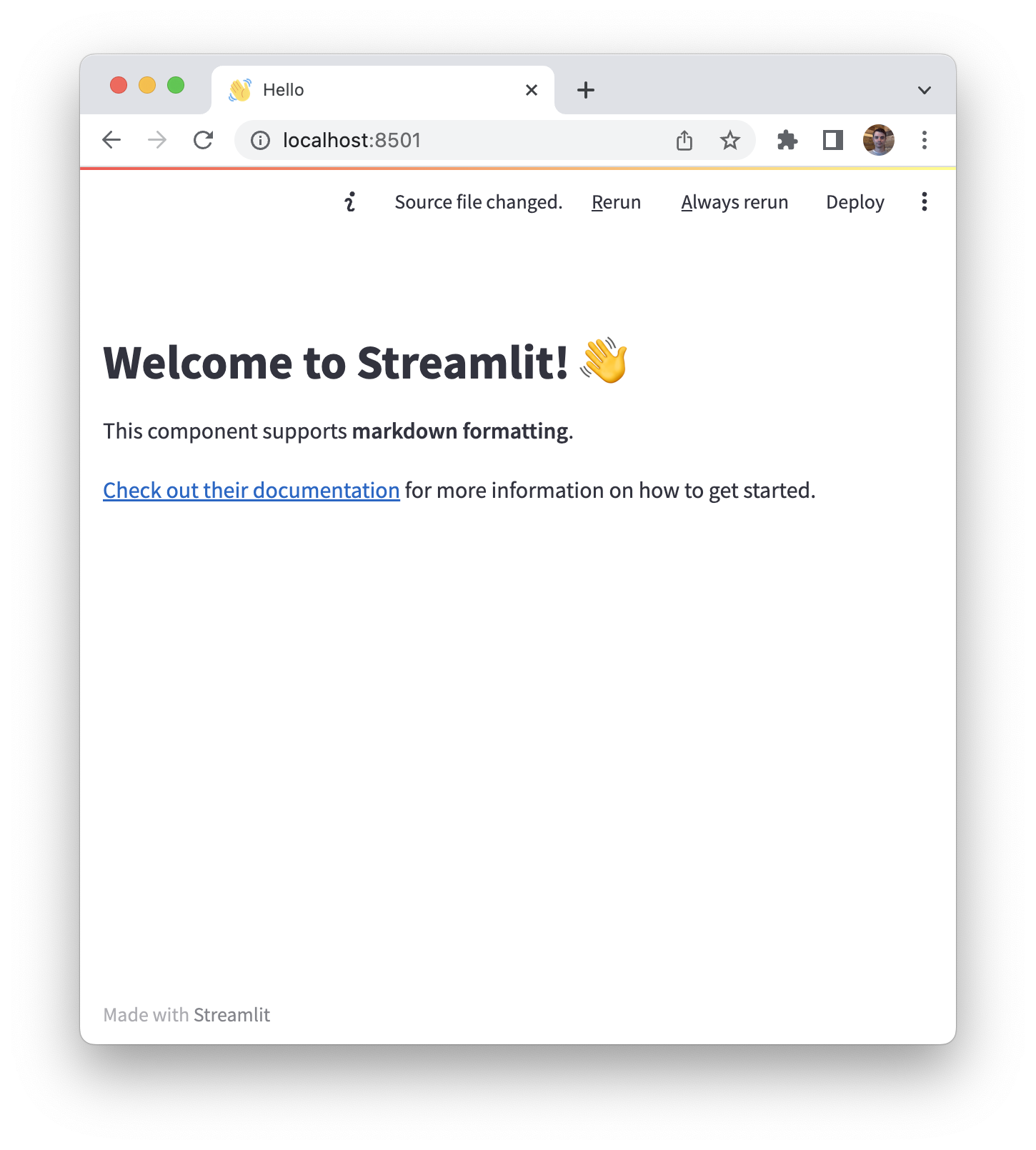
Streamlit provides methods to create Chat elements, making it trivial to build a chatbot interface.
LLM Integration with Langchain
You will write code to integrate the chatbot with a Large Language Model (LLM). At the start of the course, the AI assistant simply repeats the user input.
If you have completed the Using Neo4j with LangChain course, you will be familiar with LangChain. LangChain is an open-source framework designed to accelerate the development of LLM applications. Langchain provides a flexible base for testing LLMs and out-of-the-box chains for complex tasks.
You can use the LLM integration components with any framework that supports Python, such as Flask, FastAPI, or Django.
LLMs from OpenAI
The course contains instructions for using OpenAI’s Large Language Models.
You are not restricted to OpenAI, however. The hands-on challenges in this course are LLM-agnostic, and you are free to use any of the LLMs supported by Langchain.
Ready to get started?
In the next lesson, you will setup and run the starter project.
Summary
In this lesson, you learned about the components you will use in the course.
In the next lesson, you will setup and run the starter project.